Threads are a mechanism that permits an application to perform various tasks. The thread has its own stack but it shares the global memory of the parent process which includes initialized data, uninitialized data, and heap segments.
The memory segment sharing is shown below:
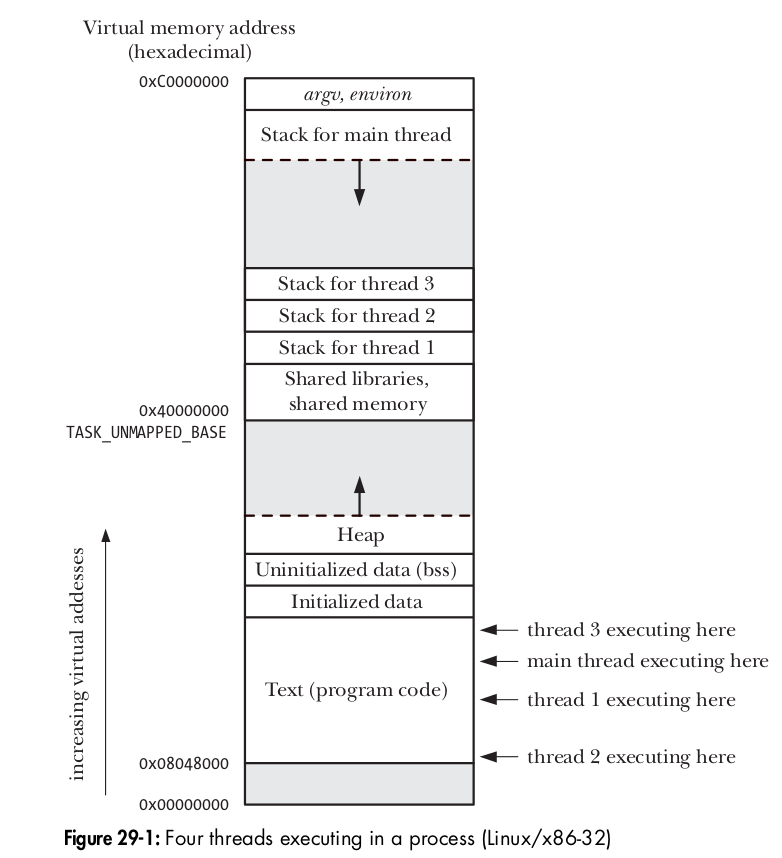
The threads spawned by a process can execute concurrently. Threads provide the following advantages with respect to a process:
- sharing information between threads is faster and easier as the data to be shared can be part of global shared variables
- Thread creation is faster than process creation. Threads are created using “clone” command and many of the process attributes that are needed to create a process are instead shared between threads. Hence, thread creation is supposedly much faster
Threads share the following with other threads spawned by the same parent
- process ID and parent ID
- process group ID and session ID
- process credentials
- open file descriptors
- locks, signals and signal masks, timers, CPU affinity, etc
Linux kernel supports POSIX threads which have ‘p’ attached at the start. Hence, a POSIX thread will be termed a “pthread”. The pthread data types are provided below:
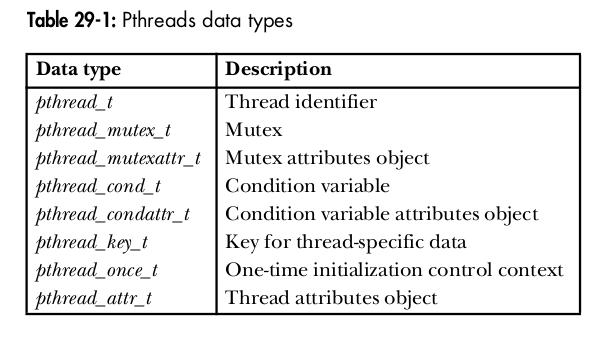
Some of the Basic APIs for pthreads are listed below
pthread API | Description |
pthread_create(pthread_t *thread, const pthread_attr_t *attr, void (start)(void *), void *arg) | create a POSIX thread |
pthread_exit(void *retval) | An API to terminate the thread. A thread can also exit if the thread just performs exit using return. The pthread_exit function terminates the calling thread and specifies a return value that can be obtained in another thread by calling “pthread_join” API |
pthread_join(pthread_t thread, void **retval) | The name is a bit misleading. The API waits for the thread identified by the “thread” parameter to terminate. if that thread ha already terminated, pthread_join returns immediately. |
pthread_detach(pthread_t thread) | Normally a thread is termed “joinable”. That is, if the thread is terminated, another thread can get the thread status by waiting on pthread_join. However, if we do not wish to know of the return status of the thread and wish the system to clean up resources as soon as thread terminates – then pthread_detach is used for the purpose |
“pthread.h” header file contains a more exhaustive listing of pthread APIs.
Pingback: FIFO Inter-processing communication in Linux User space | Hitch Hiker's Guide to Learning